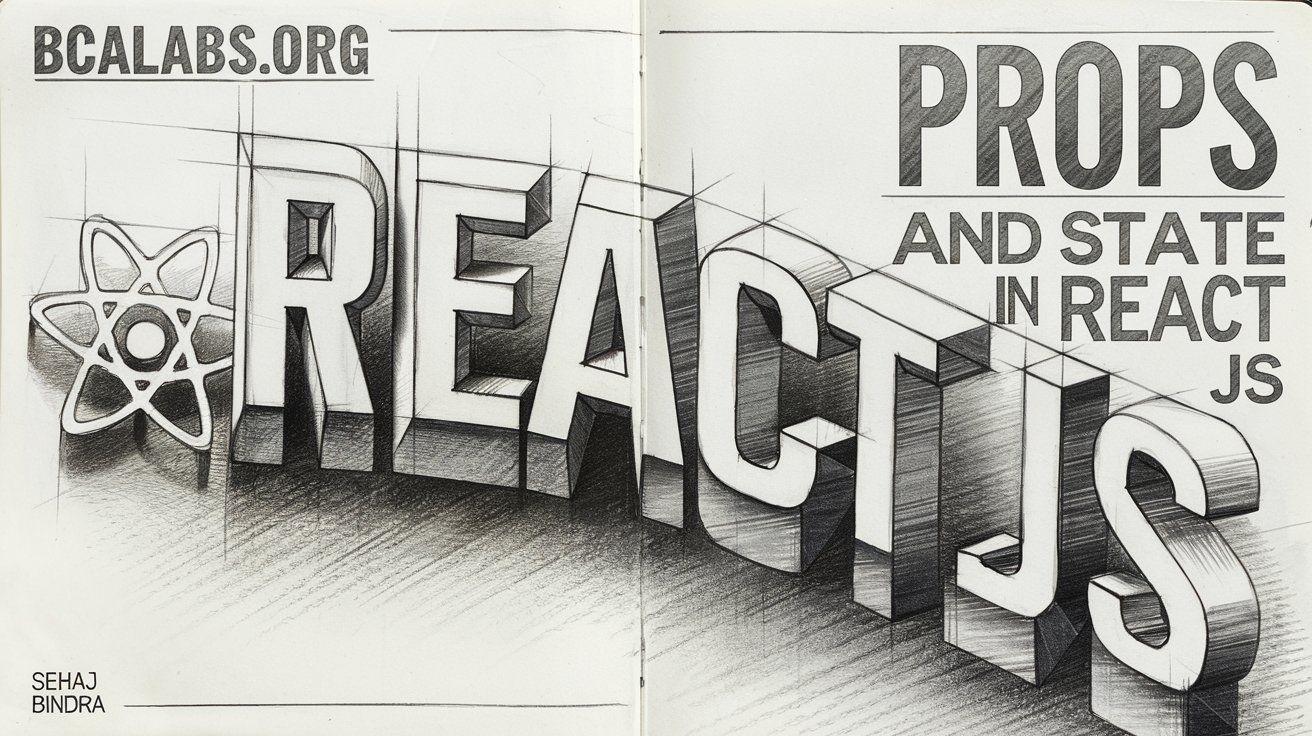
Props and state in React js | Need of Props and State in Reactjs
What are Props and State in React?
- In React, components can accept two main types of data: props and state.
- Both are used to control how components behave and render, but they serve different purposes.
Props (Properties)
- Props are read-only attributes that are passed down from parent components to child components.
- They are used to pass data and configuration to child components.
- Props are immutable, meaning they cannot be changed by the component that receives them.
Why Do We Use Props?
- Props are essential for building reusable and dynamic components.
- They allow components to remain flexible and functional by accepting input data without tightly coupling to a particular context. Here’s why we need props:
- Component Reusability: Props allow a component to be reused with different data, making it versatile for different scenarios.
- Unidirectional Data Flow: Props ensure that data flows from parent to child, maintaining a predictable data flow in the application.
- Separation of Concerns: A parent component manages the data logic, and the child component focuses on rendering and presentation, improving code organization.
Example of Props:
1function UserInfo(props) {
2 return (
3 <div>
4 <p>Name: {props.name}</p>
5 <p>Age: {props.age}</p>
6 </div>
7 );
8}
9
10// Usage
11<UserInfo name="Sehaj" age={25} />
In this case, name and age are passed to the UserInfo component as props. These values are then displayed inside p tags.
Example: Passing Props to Child Component
Let’s explore a simple example where a parent component passes a name and an age to a child component using props.
1function UserProfile(props) {
2 return (
3 <div>
4 <h1>Name: {props.name}</h1>
5 <p>Age: {props.age}</p>
6 </div>
7 );
8}
9
10function App() {
11 return (
12 <div>
13 <UserProfile name="Sehaj" age={25} />
14 <UserProfile name="John" age={30} />
15 </div>
16 );
17}
18
19// Usage
20<App />
- UserProfile Component: This component accepts two props: name and age.
- These props are used to render the user’s name and age inside the component.
- App Component:The App component renders the UserProfile component twice, passing different values for name and age props each time.
- This allows the same UserProfile component to display different user information.
- This component accepts two props: name and age. These props are used to render the user’s name and age inside the component.
Result:
- The first instance shows: "Name: Sehaj, Age: 25"
- The second instance shows: "Name: John, Age: 30"
Example : Props for Customizing Child Component Behavior
In the following example, props are used to customize the behavior of a button component.
1function Button(props) {
2 return (
3 <button onClick={props.onClick} style={{ backgroundColor: props.color }}>
4 {props.label}
5 </button>
6 );
7}
8
9function App() {
10 const handleClick = () => {
11 alert('Button Clicked!');
12 };
13
14 return (
15 <div>
16 <Button label="Click Me" color="blue" onClick={handleClick} />
17 <Button label="Submit" color="green" onClick={handleClick} />
18 </div>
19 );
20}
21
22// Usage
23<App />
Button Component: The Button component accepts three props: label, color, and onClick.
- label: The text displayed inside the button.
- color: The button's background color, passed as a style property.
- onClick: The event handler to be called when the button is clicked.
- App Component: The App component uses the Button component twice
- , passing different values for the label and color props, but the same onClick function.
Result
- The first button says "Click Me" with a blue background.
- The second button says "Submit" with a green background.
- Both buttons trigger an alert when clicked, using the same handleClick function.
State
- State is a way for components to store and manage data internally. Unlike props,
- state is mutable and can change over time, often in response to user input or other events.
- Components re-render whenever their state changes. State was primarily used in class components,
- but with the introduction of React Hooks (like useState), it can also be used in functional components.
Why Do We Need State?
State is essential for managing data that changes over time and directly affects the UI. Some common use cases for state include:
- Tracking user input: Such as text entered into a form field.
- Handling UI changes: Like toggling between light and dark mode.
- Managing loading indicators: To show the user that a process is ongoing.
- Conditionally rendering content: Based on certain events or interactions.
- Updating component data from API responses: To dynamically render new data fetched from a server.
Whenever state changes, React re-renders the component, ensuring that the UI reflects the latest state of the application. This dynamic update process is what makes state management so powerful.
We will understand this concept in detail in upcoming blogs!!
Example: Using State for a Checkout Process
A typical use case for state is showing a loading indicator when a user initiates a process, such as checking out.
Example of State in a Class-based Component:
1class Counter extends React.Component {
2 constructor(props) {
3 super(props);
4 this.state = { count: 0 };
5 }
6
7 increment = () => {
8 this.setState({ count: this.state.count + 1 });
9 };
10
11 render() {
12 return (
13 <div>
14 <p>Count: {this.state.count}</p>
15 <button onClick={this.increment}>Increment</button>
16 </div>
17 );
18 }
19}
20
21// Usage
22<Counter />
- In this example, Counter is a class-based component with internal state. The count state is initialized to 0 in the constructor.
- The increment method updates the state using this.setState(), causing the component to re-render with the new count value.
- The Increment button triggers the state change.
Example of State in a Functional Component (with Hooks)
1import React, { useState } from 'react';
2
3function Counter() {
4 const [count, setCount] = useState(0);
5
6 return (
7 <div>
8 <p>Count: {count}</p>
9 <button onClick={() => setCount(count + 1)}>Increment</button>
10 </div>
11 );
12}
13
14// Usage
15<Counter />
16
- With React Hooks, state can also be managed inside functional components. In the example above,
- useState initializes the count variable and provides a setCount function to update the state.
- Each time the button is clicked, setCount increments the count, and the component re-renders.
Differences Between Props and State
- Props: Passed from parent to child component; read-only and immutable.
- State: Managed within the component itself; can be updated dynamically, triggering a re-render.
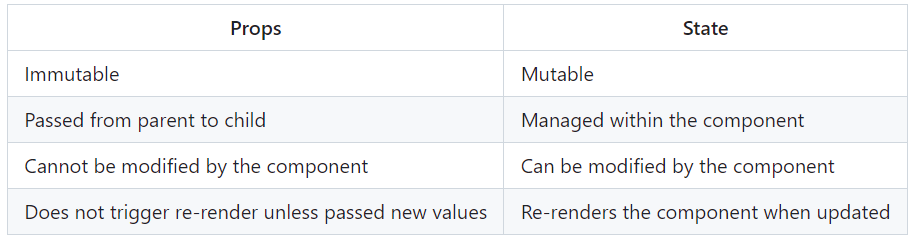
Conclusion
- Props: Passed from parent to child components, immutable, used for reusability and consistent data flow.
- State: Internal to components, mutable, used for managing dynamic, changing data.
- Props enable controlled, predictable component behavior through external inputs.
- State allows components to react to user actions and changes, updating the UI dynamically.