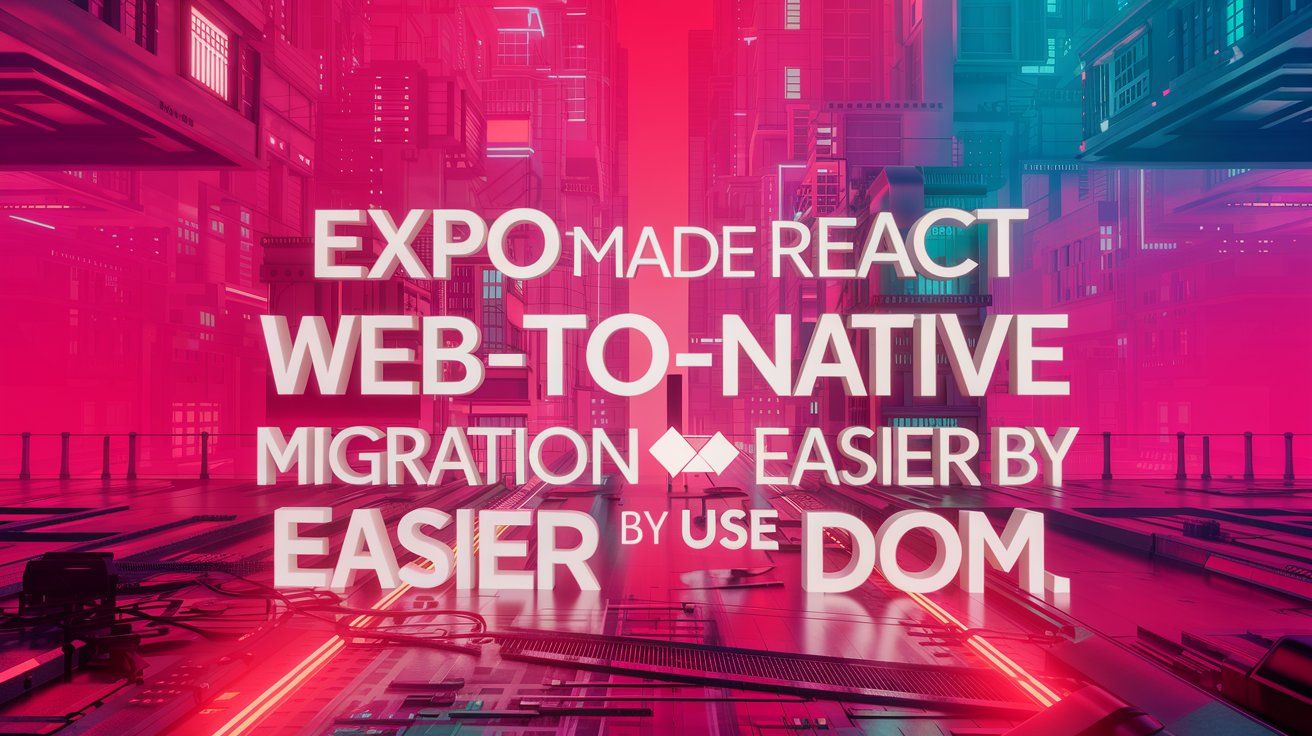
Expo Made React Web-to-Native Migration easier by "Use Dom"
Expo has long been a powerful tool for building cross-platform mobile applications.
However, transitioning from a web-based React application to a native one has often been a challenging process.
Expo Dom components, an experimental feature that promises to bridge this gap and revolutionize the way we approach React Native development.
What are Expo Dom Components?
- Expo Dom components allow developers to incrementally adopt native views in React websites without starting from scratch.
- This groundbreaking approach, introduced by Evan Bacon, the creator of Expo Router, offers a seamless way to transition from web to mobile development.
useDom Directive
- By simply adding "useDom" directive to your React components like we are doing with client components in nextjs 14 "use client", you can render web components in a native environment.
- This means you can take your existing web components and, with minimal modification, use them in a React Native application.
1'use dom';
2
3export default function DOMComponent() {
4 return <p>Hello DOM</p>;
5}
6
The Benefits of Expo Dom Components
1. Rapid Prototyping and Development
- One of the most significant advantages of Expo Dom components is the ability to quickly prototype and develop mobile applications using existing web components.
- This can dramatically reduce development time and allow for faster iteration.
2. Incremental Native Adoption
With Expo Dom components, you're not forced to rewrite your entire application for native platforms. Instead, you can:
- Start with your existing web components
- Gradually replace or optimize specific components for native performance
- Focus on areas that need the most attention for mobile user experience
3. Shared Code Base
Expo Dom components allow for a shared code base between web and mobile applications. This means:
- Changes made to components are reflected in both web and mobile versions
- Easier maintenance and consistency across platforms
- Reduced development overhead
Implementing Expo Dom Components
Setting Up Your Project
To get started with Expo Dom components, you'll need to set up your project with Expo.
Clone this Repository
OR
1npx create-expo-app expo-dom-components
After that We Have to Install Certain packages
1) react-native-webview in your project
1npx expo install react-native-webview
2) Expo Sdk 52 Canary
1npm install expo@^52.0.0-canary-20240814-ce0f7d5
After running this we have to run this command as it fix the dependencies conflict
1npx expo install --fix
then after running the above command make sure your package.json looks like this :
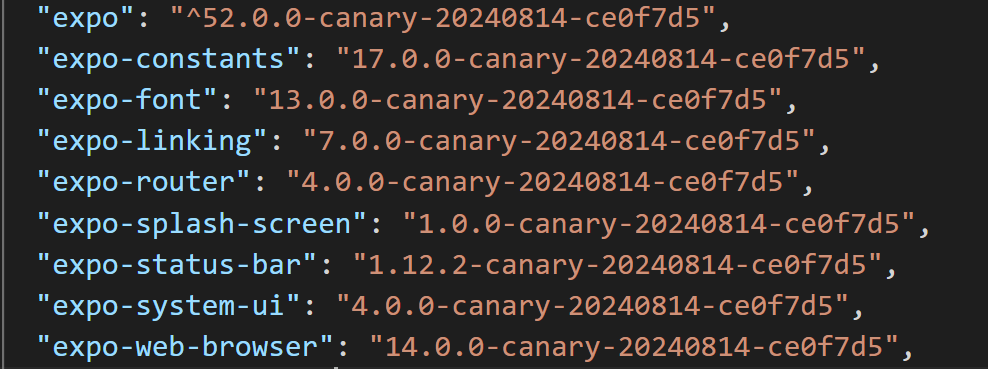
Advanced Features and Considerations
Native Functionality in Dom Components
One exciting aspect of Expo Dom components is the ability to add native functionality to your web components. For example, you could:
- Add haptic feedback to button presses
- Implement native notifications
- Utilize device-specific features
Data Flow and Props
When working with Expo Dom components, it's important to note that:
- You can send data to Dom components through serializable props
- Props are sent asynchronously
- Complex data structures cannot be sent as props
1import DOMComponent from './my-component';
2
3export default function App() {
4 return <DOMComponent hello={'world'} />;
5}
6
You have to add "use dom" directive at the top file to make dom components
1'use dom';
2
3export default function DOMComponent({ hello }: { hello: string }) {
4return <p>Hello, {hello}</p>;
5}
Native Actions
- Expo Dom components allow you to send TypeScript native functions to Dom components by passing asynchronous functions.
- This opens up a world of possibilities for integrating native functionality into your web components.
1import DomComponent from './my-component';
2
3export default function App() {
4 return (
5 <DomComponent
6 hello={(data: string) => {
7 console.log('Hello', data);
8 }}
9 />
10 );
11}
12
1'use dom';
2
3export default function MyComponent({ hello }: { hello: (data: string) => Promise<void> }) {
4 return <p onClick={() => hello('world')}>Click me</p>;
5}
6
Limitations and Considerations
While Expo Dom components offer exciting possibilities, it's important to keep in mind:
- Expo Dom Components are an experimental feature, and as such, their functionality may evolve over time.
- For production-ready applications, using web views directly is still recommended
- There may be performance considerations when heavily relying on Dom components because they only support standard JavaScript, which is slower to parse and start up than optimized Hermes bytecode.
For In Depth Read refer this blog by expo
Conclusion
Expo Dom components represent a significant step forward in React Native development.
By allowing developers to incrementally adopt native views and seamlessly transition between web and mobile development,
this approach has the potential to streamline the development process and make cross-platform development more accessible than ever before.