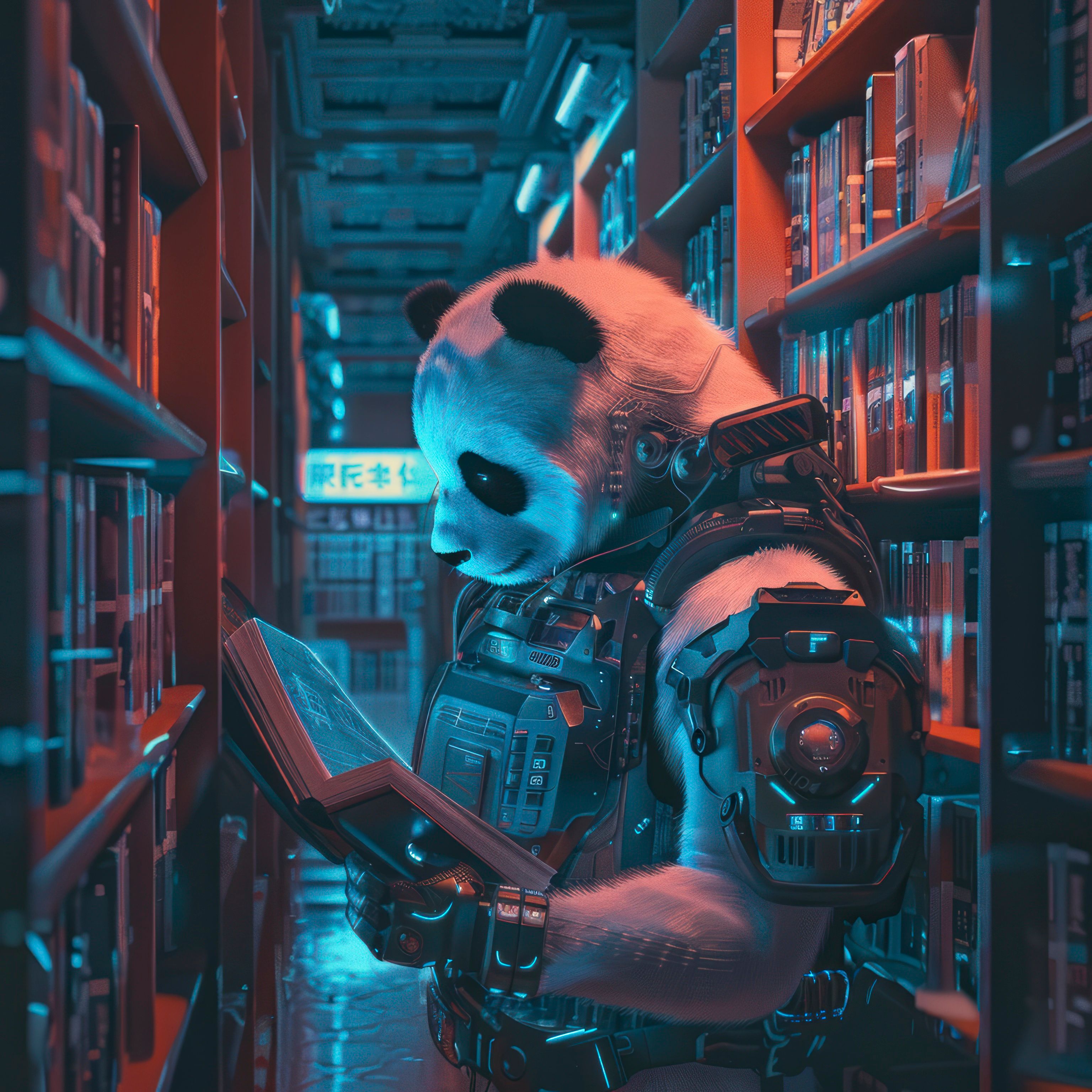
Why Python Is Required for Data Analyst
Python is an awesome choice for data analysis, and here’s why
- Easy to Learn and Use: Python is simple and easy to pick up.
- Its clean and straightforward syntax lets you write code quickly and focus more on analyzing data than figuring out the programming language.
- Large Community Support: Python has a huge and active community. Many resources like libraries, tutorials, and forums help you with any data analysis task.
- Rich Ecosystem of Libraries and Tools: Python comes with a wide range of powerful libraries and tools like NumPy, Pandas, Matplotlib, and Scikit-learn.
- These make it easy to handle big datasets, perform complex data tasks, visualize data, and even build machine learning models.
- Works Well with Other Languages: Python can easily work with other programming languages like R and C++.
- This makes it super flexible for various tasks.
- High-Level Programming: Python takes care of low-level details like memory management, so you can concentrate on analyzing your data.
IMPORTANT TOPICS TO BE COVERED IN PYTHON
Understanding Basics
- Python syntax
- Variables, and basic data types.
Control flow with if statements, loops, - functions
Data Structures
- Explore Lists, Tuples, Sets, and Dictionaries.
- How to manipulate and iterate through these data structures.
Object-Oriented Programming (OOP)
- Basics of OOP, including classes and objects.
- constructors and destructors
- Inheritance
- Polymorphism
- Encapsulation
- Abstraction
File Handling
- How do you read from and write to files in Python.
- Working with different file formats(CSV, JSON).
1# Writing to a file
2try:
3 with open("example.txt", "w") as file: # "w" mode means write (creates or truncates)
4 file.write("Hello, world!\n")
5 file.write("This is an example of file handling in Python.")
6except IOError as e:
7 print("Error writing to file:", e)
8
9# Reading from a file
10try:
11 with open("example.txt", "r") as file: # "r" mode means read
12 content = file.read()
13 print("File content:\n", content)
14except IOError as e:
15 print("Error reading from file:", e)
16
Exceptional handling
How to handle the exceptions and errors in our code, using TRY, EXCEPT, FINALLY blocks.
1try:
2 # Code that may raise an exception
3 numerator = 10
4 denominator = 0
5 result = numerator / denominator
6 print("The result is:", result)
7except ZeroDivisionError as e:
8 # Handle the specific exception
9 print("Error: Cannot divide by zero.")
10 print("Exception message:", e)
11except Exception as e:
12 # Handle any other exceptions
13 print("An unexpected error occurred:", e)
14else:
15 # Code that runs if no exception occurred
16 print("Division was successful.")
17finally:
18 # Code that always runs, regardless of an exception
19 print("Execution of the try-except block is complete.")
20
Modules and Packages
- Creating and Importing modules.
- Organizing Codes into modules.
Important libraries
NUMPY
Think of NumPy as the foundation of your data science skyscraper. It's all about working with large, multi-dimensional arrays and matrices, making number crunching super efficient.
Whether you’re doing complex math or just basic calculations, NumPy is your go-to buddy.
How to install Numpy
Make sure Python is installed on your system. You can check by opening a terminal (or command prompt) and typing
1pip --version
2
- Once you have Python and pip installed, you can install NumPy using pip. Open your terminal (or command prompt) and type.
1pip install numpy
- To ensure NumPy was installed correctly, you can open a Python interpreter (by typing python or python3 in your terminal) and then try importing.
1import numpy as np
Example
1import numpy as np
2
3# Create a 1D array
4array_1d = np.array([1, 2, 3, 4, 5])
5print("1D Array:", array_1d)
6
7# Create a 2D array
8array_2d = np.array([[1, 2, 3], [4, 5, 6]])
9print("2D Array:\n", array_2d)
10
11# Element-wise addition
12added_array = array_1d + 10
13print("Added Array:", added_array)
14
15# Element-wise multiplication
16multiplied_array = array_1d * 2
17print("Multiplied Array:", multiplied_array)
18
19# Accessing elements
20first_element = array_1d[0]
21print("First Element:", first_element)
22
23# Slicing arrays
24slice_array = array_1d[1:4]
25print("Sliced Array:", slice_array)
26
27# Generate an array of zeros
28zeros_array = np.zeros((3, 3))
29print("Zeros Array:\n", zeros_array)
30
31# Generate an array of ones
32ones_array = np.ones((2, 4))
33print("Ones Array:\n", ones_array)
34
35# Generate an identity matrix
36identity_matrix = np.eye(3)
37print("Identity Matrix:\n", identity_matrix)
38
39# Calculate the mean of an array
40mean_value = np.mean(array_1d)
41print("Mean Value:", mean_value)
42
43# Calculate the sum of elements in an array
44sum_value = np.sum(array_1d)
45print("Sum Value:", sum_value)
46
47# Calculate the standard deviation of an array
48std_dev = np.std(array_1d)
49print("Standard Deviation:", std_dev)
50
51# Matrix multiplication
52matrix_a = np.array([[1, 2], [3, 4]])
53matrix_b = np.array([[5, 6], [7, 8]])
54matrix_product = np.dot(matrix_a, matrix_b)
55print("Matrix Product:\n", matrix_product)
56
57# Matrix transpose
58transpose_matrix = np.transpose(matrix_a)
59print("Transpose of Matrix A:\n", transpose_matrix)
60
PANDAS
Imagine having a magical toolbox for all your data needs! Pandas is just that—it makes handling data a breeze with its Data Frames and Series.
Like a pro, you can read, write, clean, transform, and analyze data. Need to filter, group, or aggregate data? Pandas has got you covered!
How to install Pandas
Once you have Python and pip installed, you can install Pandas using pip. Open your terminal (or command prompt) and type.
1pip install pandas
To ensure Pandas was installed correctly, you can open a Python interpreter (by typing python or python3 in your terminal) and then try importing Pandas
1import pandas as pd
2print(pd.__version__)
3
Example
1import pandas as pd
2
3# Create a DataFrame from a dictionary
4data = {
5 'Name': ['Alice', 'Bob', 'Charlie', 'David', 'Eva'],
6 'Age': [24, 27, 22, 32, 29],
7 'City': ['New York', 'Los Angeles', 'Chicago', 'Houston', 'Phoenix']
8}
9
10df = pd.DataFrame(data)
11print("DataFrame:\n", df)
12
13# Save the DataFrame to a CSV file
14df.to_csv('data.csv', index=False)
15
16# Read data from the CSV file
17df_from_csv = pd.read_csv('data.csv')
18print("\nDataFrame from CSV:\n", df_from_csv)
19
20# Select specific columns
21selected_columns = df[['Name', 'City']]
22print("\nSelected Columns:\n", selected_columns)
23
24# Filter rows based on a condition
25filtered_rows = df[df['Age'] > 25]
26print("\nFiltered Rows (Age > 25):\n", filtered_rows)
27
28# Group data by 'City' and calculate the mean age
29grouped_data = df.groupby('City')['Age'].mean()
30print("\nGrouped Data (Mean Age by City):\n", grouped_data)
31
32# Add a new column with missing values
33df['Salary'] = [50000, 60000, None, 80000, None]
34print("\nDataFrame with Missing Values:\n", df)
35
36# Fill missing values with a specific value
37df_filled = df.fillna(0)
38print("\nDataFrame with Missing Values Filled:\n", df_filled)
39
40# Drop rows with missing values
41df_dropped = df.dropna()
42print("\nDataFrame with Missing Values Dropped:\n", df_dropped)
43
MATPLOTLIB and SEABORN
Ready to turn your data into stunning visual stories? Matplotlib lets you create all kinds of charts and plots, from static to interactive.
Seaborn takes it up a notch with beautiful and easy-to-create statistical graphics. Your data will not only be informative but also visually appealing!
How to import Matplotlib and seaborn
Open your terminal (or command prompt) and type the following commands
1import matplotlib.pyplot as plt
2import seaborn as sns
3
- To make sure the libraries are working correctly
1import matplotlib.pyplot as plt
2import seaborn as sns
Example
1import matplotlib.pyplot as plt
2import seaborn as sns
3import numpy as np
4import pandas as pd
5
6# Create a sample DataFrame
7np.random.seed(0)
8data = pd.DataFrame({
9 'Category': np.random.choice(['A', 'B', 'C'], size=100),
10 'Value1': np.random.randn(100),
11 'Value2': np.random.rand(100) * 100
12})
13
14# Line plot using Matplotlib
15plt.figure(figsize=(10, 5))
16plt.plot(data['Value1'], label='Value1')
17plt.plot(data['Value2'], label='Value2')
18plt.title('Line Plot using Matplotlib')
19plt.xlabel('Index')
20plt.ylabel('Values')
21plt.legend()
22plt.show()
23
24# Scatter plot using Matplotlib
25plt.figure(figsize=(10, 5))
26plt.scatter(data['Value1'], data['Value2'], c='blue', alpha=0.5)
27plt.title('Scatter Plot using Matplotlib')
28plt.xlabel('Value1')
29plt.ylabel('Value2')
30plt.show()
31
32# Bar plot using Seaborn
33plt.figure(figsize=(10, 5))
34sns.barplot(x='Category', y='Value2', data=data, ci=None)
35plt.title('Bar Plot using Seaborn')
36plt.xlabel('Category')
37plt.ylabel('Value2')
38plt.show()
39
40# Pair plot using Seaborn
41sns.pairplot(data, hue='Category')
42plt.suptitle('Pair Plot using Seaborn', y=1.02)
43plt.show()
44
45
46
47
48
49
50
SKILERN
Scikit-learn is like a treasure chest full of powerful tools for data mining and analysis.
Whether you’re into classification, regression, clustering, or reducing dimensions, this library has it all.
Plus, it helps you evaluate models, tune hyperparameters, and pick the best models effortlessly.
you can install Scikit-learn using pip. Open your terminal (or command prompt) and type
1pip install scikit-learn
2
To ensure Scikit-learn was installed correctly, you can open a Python interpreter (by typing python or python3 in your terminal) and then try importing Scikit-learn
1import sklearn
2print(sklearn.__version__)
3
Database connectivity
- connection to databases (eg.SQLite,MySQL OR PostgreSQL)
- Performing CRUD operations.
General Steps for Any Database
- Install the appropriate library/package for your database.
- Establish a connection using the appropriate connection string or parameters (host, user, password, database name, etc.).
- Create a cursor object to execute SQL queries.
- Execute your SQL queries (e.g., create tables, insert data, query data).
- Commit your changes if necessary.
1pip install mysql-connector-python /*install my sql connector*/
2
3import mysql.connector
4
5# Connect to the database
6conn = mysql.connector.connect(
7 host='localhost',
8 user='yourusername',
9 password='yourpassword',
10 database='yourdatabase'
11)
12
13# Create a cursor object
14cursor = conn.cursor()
15
16# Example query: create a table
17cursor.execute('''CREATE TABLE IF NOT EXISTS users (id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(255), age INT)''')
18
19# Commit changes
20conn.commit()
21
22# Close the connection
23conn.close()
24
Google colab
Google colab is a free cloud service provided by Google that allows you to write and execute Python code in a web-based interactive environment.
How to use Google colab
What is EDA ?
Exploratory Data Analysis (EDA) is a crucial and exciting step in the data analysis process where you get to dive deep into your dataset to uncover its secrets.
The goal of EDA is to understand your data, spot patterns, detect anomalies, test hypotheses, and validate assumptions—all with the power of summary statistics and eye-catching visualizations.
Steps of EDA in Python
- Loading Data: Importing the dataset into a Pandas DataFrame.
- Understanding Data: Getting basic information about the dataset.
- Data Cleaning: Handling missing values, duplicates, and errors.
- Data Transformation: Transforming data types, creating new features.
- Data Visualization: Visualizing data to uncover underlying patterns.
- Summary Statistics: Calculating descriptive statistics to summarize the data.
Outliers: Detect, Analyze, and Harness Their Impact
Outliers are data points that differ significantly from other observations in a dataset.
They can be unusually high or low values and may indicate variability in the data, errors in data collection or entry, or the presence of a novel phenomenon.
Importance of Identifying and Treating Outliers in Data Analytics
Data Quality and Integrity
- Error Detection Outliers may indicate errors or inaccuracies, crucial for data quality.
- Preprocessing Addressing outliers is key in data cleaning for reliable analysis.
Impact on Statistical Analysis
- Distorted Statistics Outliers can skew measures like mean and variance, misleading summaries.
- Assumption Violations They can violate normality assumptions in statistical tests and models.
Effect on Predictive Models
- Model Performance Outliers can cause overfitting or underfitting, affecting accuracy.
- Bias and Variance Proper treatment helps balance model bias and variance.
Insight and Decision Making
- Misleading Insights Outliers can distort trends and lead to incorrect conclusions.
- Opportunities They may reveal unique opportunities, such as fraud detection or new customer segments.
Understanding Distribution Characteristics
- Skewness and Kurtosis Outliers affect distribution shape, indicating potential anomalies.
Resource Allocation
- Efficiency Proper handling optimizes computational resource use.
Compliance and Reporting
- Regulatory Needs Necessary for compliance in sectors like finance and healthcare.
Treatment of Outliers in Data Analytics
- Removing Outliers: Remove if due to errors or irrelevant, but verify they aren't significant events.
- Transforming Data: Use log or Box-Cox transformations to reduce outlier impact.
- Capping/Flooring: Limit extreme values to reduce influence (Winsorizing).
- Handling Separately: Analyze outliers separately if they represent distinct phenomena.
- Robust Statistics: Use median-based measures or robust methods.
- Machine Learning Approaches: Apply anomaly detection models to identify and handle outliers.
- Imputation: Replace outliers with plausible estimates (e.g., median).
Some free Resource to learn
Websites to get Dataset for practice
Conclusion
By mastering these Python concepts and libraries, data analysts can efficiently manipulate and analyze data, create insightful visualizations, apply machine learning techniques, and derive valuable insights from their datasets.