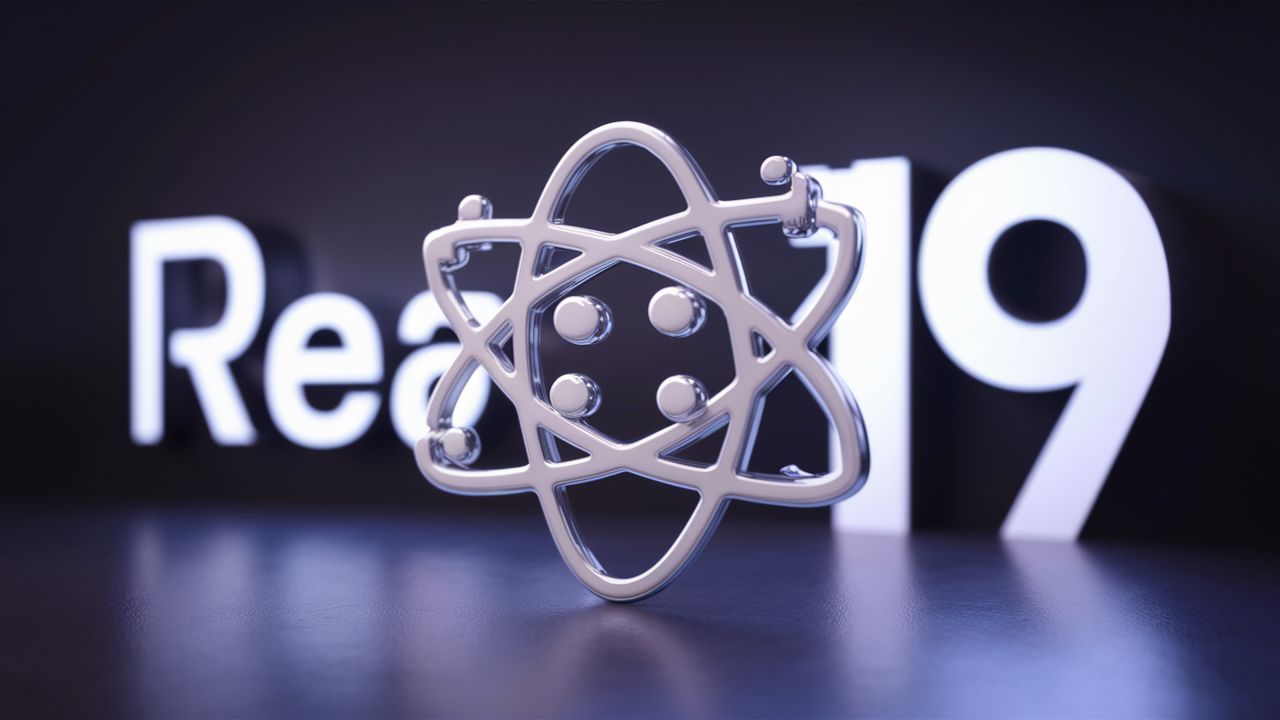
React 19 : React Compiler, Actions and React Canary
React, the JavaScript library for creating user interactions, is evolving and the next React 19 brings major updates such as React Compiler, Server Components, Actions, React Canary and more to improve performance and productivity.
React Compiler: Automating Memoization for Enhanced Performance
In React 19, the React compiler takes center stage, moving from a research project to an electronic production tool like instagram.com in Meta.
What is Memoization?
- Memoization in React optimizes component performance by caching and reusing the results of expensive calls when the same input is encountered again.
- In simpler terms, it helps prevent unnecessary re-execution of computations.
- You can achieve memoization in React using the React.memo higher-order component or the useMemo hook.
React.memo: is a higher-order component that you can wrap around a functional component.
1import React from 'react';
2
3const MyComponent = React.memo((props) => {
4 // Your component logic here
5});
6
7export default MyComponent;
8
useMemo Hook: is a hook that allows you to memoize the result of a function or a computation.
1import React, { useMemo } from 'react';
2
3const MyComponent = ({ data }) => {
4 const memoizedResult = useMemo(() => {
5 // Expensive computation based on data
6 return someResult;
7 }, [data]); // Only recompute if 'data' changes
8};
9
10export default MyComponent;
To prevent the above Manual memoization the React 19 version introduces the React compiler.
Need For React Compiler
The primary goal is to optimize re-renders by automating manual memoization tasks, such as using useMemo, useCallback, and memo APIs.
- Code Clutter: The React Compiler allow React to automatically re-render the appropriate parts of the UI when state changes without compromising the simplicity of React's core mental model.
- This eliminates the need for developers to manually handle re-renders, reducing code complexity.
- Improved Debugging: By automating memoization and optimizing re-renders, the React Compiler contributes to improved error handling and debugging.
How it Works
- The compiler aims to automatically re-render specific parts of the UI when state changes, adhering to React's core mental model of UI as a simple function of state.
- By modeling both JavaScript and React rules, the compiler ensures safe and efficient code compilation.
- It detects deviations from React's rules and either compiles the code where safe or skips compilation if not.
Actions: Simplifying Data Handling Across Client and Server
Actions in React 19 provide a streamlined approach to handling data submissions, whether on the client or server.
Initially explored for server actions, the concept expanded to support data handling in client-only applications as well.
Key Features
- Client-Side or Server-Side Actions: Define actions using standard JavaScript on the client or leverage the 'use server' directive for server-side actions.
- Asynchronous Support: Actions support both synchronous and asynchronous functions, enabling developers to manage data submission seamlessly.
- Optimistic Updates: Introducing the useOptimistic hook allows developers to apply temporary updates optimistically, reverting them if the final state commits successfully.
Example
1form action={search}>
2 <input name="query" />
3 <button type="submit">Search</button>
4</form>
React Canary
React Canary serves as an avenue for developers to adopt stable features before their official release.
This approach allows the community to engage in the development process, providing feedback on features as they approach finalization.
Features in Canary
- React Server Components
- Asset Loading
- Document Metadata
- Actions
- This includes directives like "use client" and "use server," enhancing full-stack React frameworks.
React Server Components
React Server Components enable server-side rendering, allowing components to be rendered on the server and seamlessly integrated with client-side React components.
Enhanced Performance: By offloading computation to the server, React Server Components contribute to faster initial page loads, improving performance, and enhancing the user experience.
Asset Loading
Integration with Suspense: Asset Loading in React 19 integrates with Suspense, synchronizing the loading lifecycle of resources like stylesheets, fonts, and scripts with React.
Document Metadata
Built-in Support: React 19 introduces built-in support for rendering <title>, <meta>, and metadata <link> tags anywhere in the component tree.
React 19: The Next Major Version
- With React 19, major improvements are introduced, aligning with the philosophy of a single set of APIs working across all platforms and environments.
- The version incorporates long-requested enhancements, including support for Web Components.
Conclusion
- As React 19 approaches its release, the React ecosystem is set to receive substantial upgrades.
- From the revolutionary React Compiler to the streamlined Actions and the community-driven React Canary channel, developers can anticipate a more efficient and versatile development experience.
- Stay tuned for more updates as React 19 unfolds its potential in the coming months.