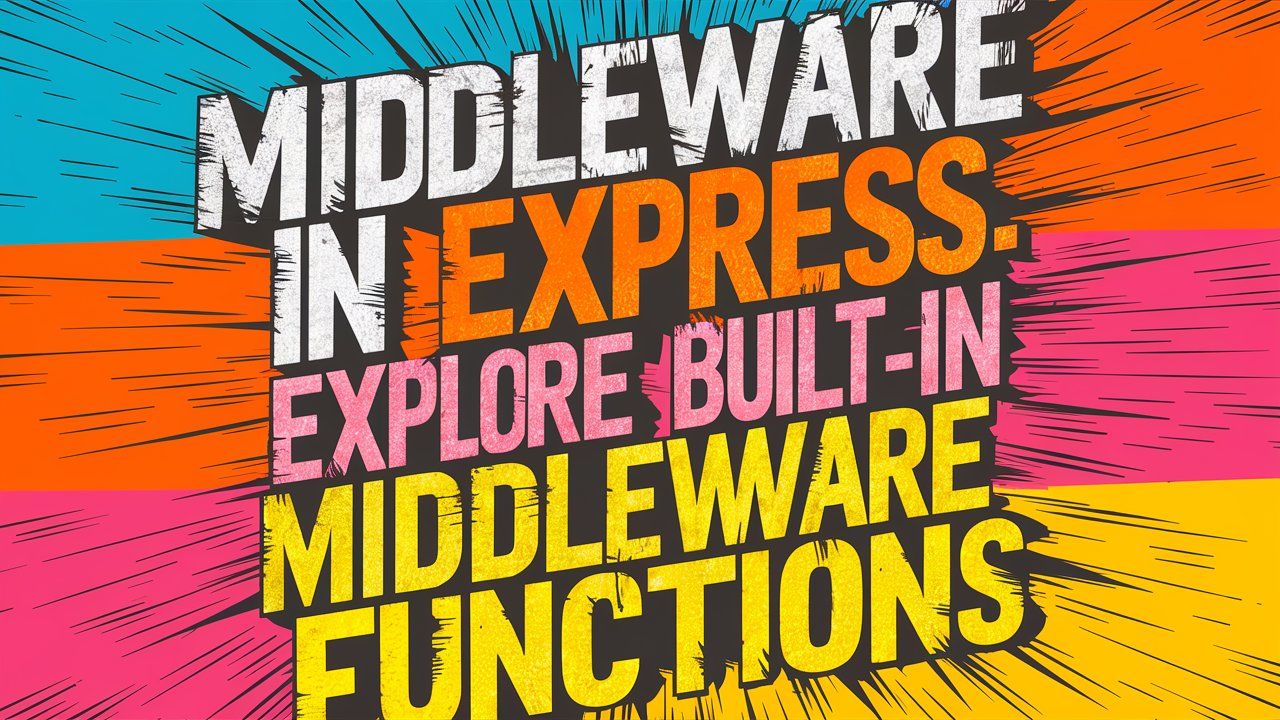
Middleware in Express: Explore built-in middleware functions
Rome wasn't built in a day... and the same goes for our first great express application! In today's article, we will learn about middleware, a crucial part of any express application.
Alright then, let's start!
What is a Middleware?
Let's understand what is a middleware using simple terminology, of an office environment.
Let's make the following assumptions:
- You are the boss, and have an assistant
- You have ordered one of your employee to get you some files
- Now, what happens when your employee is done collecting the files? He comes to your assistant and hands over the files.
- Your assistant, checks if all the files are as per your demands, if they do, you get them served on your desk.
- And in the off chance, they are not up to the demands, they are returned back to the employee.
In this case, you(the boss) were the server... your assistant was the middleware and your employee, was a client device sending a request. So in basic terms, a middleware is a piece code that performs middle level validation and checks, which means:
- When you make a request to a server, it is most likely to be intercepted by a middleware of some sort.
- The middleware will then perform the required checks/modification
- And as a green light, it will call the default next() function(more on that later) to further process the request.
Why should we use Middleware?
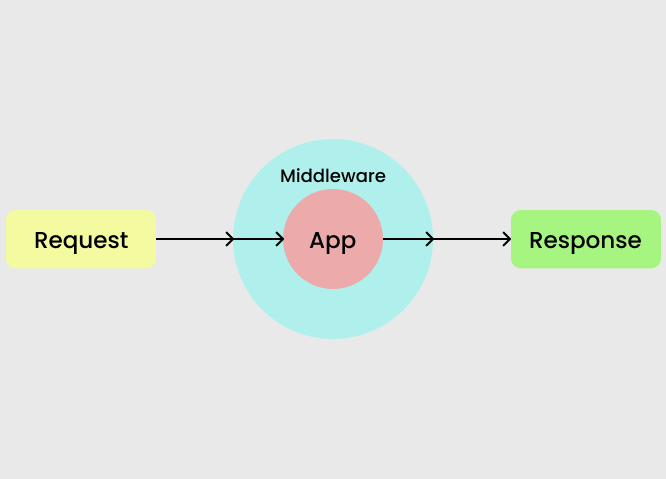
If you look at this image closely, you will see that each request, before coming in contact with the application, first goes to the middleware. Middleware(s) are often used for the following purposes:
- Formatting the incoming request to match the application standards
- Authentication and Authorization for protected API endpoints
- Terminating the req-res cycle in case of invalid requests. This helps in preventing server crashes.
- Error handling can also be done using middleware(s)
You would not want unauthorized access or invalid requests to take a toll on your server right? In that case, it's best to use suitable middleware(s). Let's talk about some common built-in middleware(s).
Built-in Middleware by Express
Express offers us some built-in middleware functions that we can use out of the box to make our lives easier! 2 of the most commonly used middleware functions are:
- JSON: This middleware is used to enable JSON data parsing for the application.
- Any incoming request, is parsed with JSON payload. To use it, just add: app.use(express.json()) to your server's entry point.
- STATIC: This middleware is used to server static files directly from your server. To do so, you can add: app.use(express.static('<directory_name>')) to your server's entry point.
But what about custom middleware? Worry not, because express supports custom middleware too! If you can't find a third-party middleware that suits you need, create your own!
You can create your very own middleware and then place it right before the actual route handler to make use of. Something liek this:
1// routes.js
2app.get("/info", <your_custom_middleware>, (req, res) => {...})
Let's Code!
- Setup a minimal express application
1mkdir express-middleware-demo
2cd express-middleware-demo
3npm init -y
4npm install express
5
- Create the entry point(index.js)
1const express = require('express');
2const app = express();
3const port = 3000;
4
5// Define middleware
6app.use((req, res, next) => {
7 console.log('Middleware 1: Request received');
8 next(); // Pass the control to next function in call stack
9});
10
11app.use((req, res, next) => {
12 console.log('Middleware 2: Processing request');
13 next();
14});
15
16// Define a route
17app.get('/', (req, res) => {
18 res.send('Hello, world!');
19});
20
21// Start the server
22app.listen(port, () => {
23 console.log(`Server is running on http://localhost:${port}`);
24});
25
- Start the server, and make a request
1node index.js
In this article, I will be using Postman's VS Code extension, which can be found here. Install it, and log in to make requests.
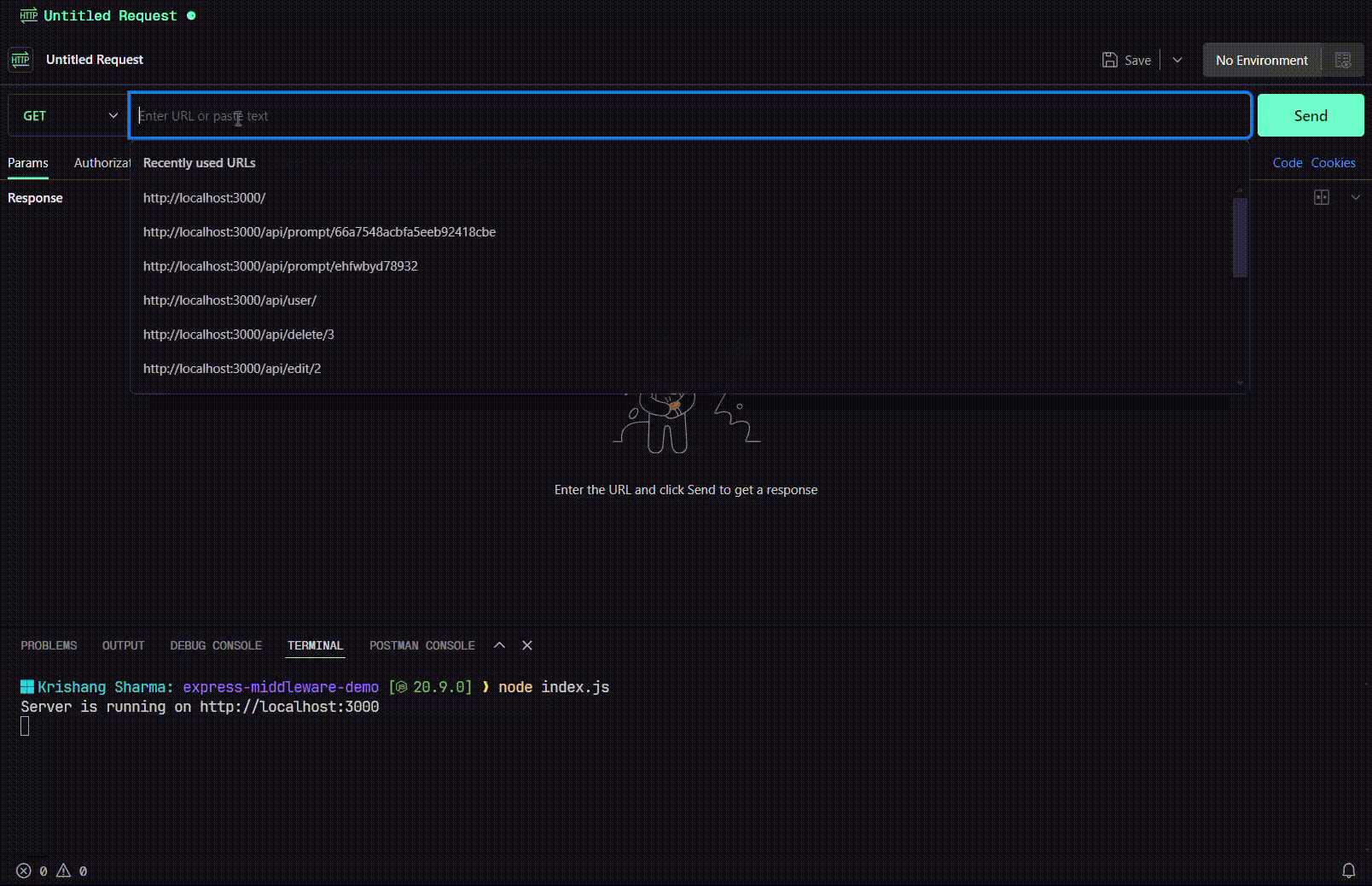
That's it! You have created custom middleware for your express application! Of course, these are just the basics, and we will increase the level once we have completed the fundamentals.
Once we get introduced to Database integration(SPOILER: next blog is about DB integration!), we will create a full fledged API with custom middleware, authentication and what not!
Until then, keep practicing what we've done till now, and I'll catch you in the next one!👋